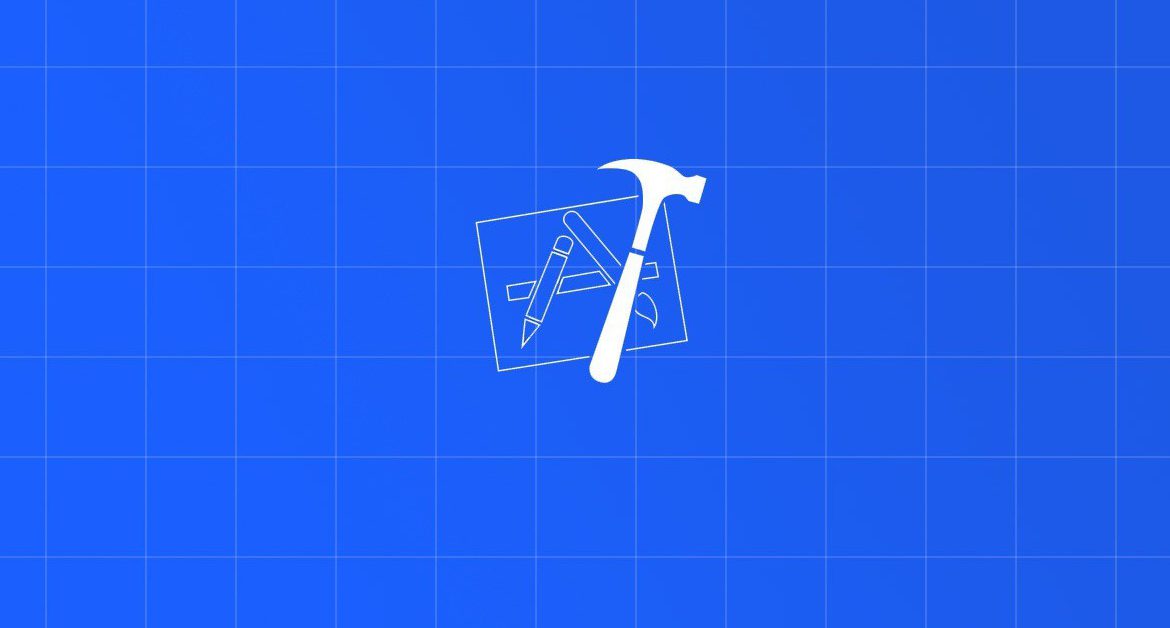
Contents
Chào bạn, bài viết hôm nay sẽ là một tutorial nhỏ hướng dẫn về Swipe Actions trong UITableViewCell.
Nếu bạn chưa biết viết TableView thì có thể xem qua các link sau:
Chúng ta quay lại chủ đề chính của bài viết. Như bạn đã biết đôi lúc chúng ta cần nhiều hơn các sự kiện cho một cell của TableView. Nhưng mà bạn chỉ có didSelectedCellForRow
, quá ít cho cuộc tình. Và nhiều khi, giao diện của bạn lại không đủ chỗ cho một loạt các button.
Giải pháp sẽ là dùng Swipe Actions trong UITableViewCell, để hiện ra các tính năng ẩn dưới Cell.
Chuẩn bị
- iOS 13
- Xcode 11.0
- Swift 5.1
Để bắt đầu, bạn chỉ cần tạo 1 project với iOS và Swift là được. Không cần quá công phu. Sau đó bạn Tạo một UITableView đơn giản là OKE.
1. Swipe Action trong UITableViewCell
Trước tiên, chúng ta có các loại sự kiện Swipe Action trong UITableViewCell.
1.1. Edit Row
Swipe để hiển thị chế độ edit
của một cell. Thường sử dụng là delete
Chúng được sử dụng thông qua việc khai báo 2 function của protocol UITableViewDataSource và Deleagate. Phòng khi bạn đã quên thì code của nó như sau:
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath { // Return YES if you want the specified item to be editable. return YES; } // Override to support editing the table view. - (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath { if (editingStyle == UITableViewCellEditingStyleDelete) { //add code here for when you hit delete } }
Nhiệm vụ của bạn rất đơn giản là dùng tay vuốt từ phải sang trái trên cell, thế là nút delete
sẽ hiện ra. Nếu lỡ tay vuốt mạnh quá thì nó sẽ đi luôn. Và xem như đã thực hiện 1 tác vụ.
Và khi bạn muốn nhiều hơn 1 cái nút thì Apple cũng không phụ lòng bạn. Nhưng chúng ta lại chia ra 2 thời kỳ:
1.2. Edit Actions (iOS 8 )
Với loại này, bạn có thể tạo một cách nhanh chóng, thậm chí khá là đơn giản nữa. Bạn sẽ sử dụng class UITableViewRowAction
, để khai báo các action mà bạn cần thêm vào.
Xem code ví dụ sau:
override func tableView(_ tableView: UITableView, editActionsForRowAt indexPath: IndexPath) -> [UITableViewRowAction]? { let deleteTitle = NSLocalizedString("Delete", comment: "Delete action") let deleteAction = UITableViewRowAction(style: .destructive, title: deleteTitle) { (action, indexPath) in self.dataSource?.delete(at: indexPath) } let favoriteTitle = NSLocalizedString("Favorite", comment: "Favorite action") let favoriteAction = UITableViewRowAction(style: .normal, title: favoriteTitle) { (action, indexPath) in self.dataSource?.setFavorite(true, at: indexPath) } favoriteAction.backgroundColor = .green return [favoriteAction, deleteAction] }
Kết quả ra như sau:
Cũng đẹp phải không nào, nhưng mà tiếc là iOS 8 đã quá cũ rồi. Chúng ta lại tiếp tục sang cách mới hơn xí.
1.3. Swipe Actions (iOS 11)
Một sự cải tiến lớn hơn nhiều, giúp bạn có thể vuốt cả 2 hướng. Với:
trailing
là từ phải sang tráileading
là từ trái sang phải
Các class liên quan tới:
UIContextualAction
giúp custom các action. Bạn có thể handler trực tiếp, thêm các ảnh và màu sắcUISwipeActionsConfiguration
giúp nhóm các actions lại và return về cho Tableview.
Các function bạn cần handle như sau:
// trailing func tableView(_ tableView: UITableView, trailingSwipeActionsConfigurationForRowAt indexPath: IndexPath) -> UISwipeActionsConfiguration? { //.... } // leading func tableView(_ tableView: UITableView, leadingSwipeActionsConfigurationForRowAt indexPath: IndexPath) -> UISwipeActionsConfiguration? { //.... }
2. Simple Action
Tiếp theo, chúng ta bắt tay vào tạo 1 swipe action đơn giản nào. Đầu tiên bạn implement thêm function trailingSwipeActionsConfigurationForRowAt
. Xem đoạn code sau:
func tableView(_ tableView: UITableView, trailingSwipeActionsConfigurationForRowAt indexPath: IndexPath) -> UISwipeActionsConfiguration? { // delete let delete = UIContextualAction(style: .normal, title: "Delete") { (action, view, completionHandler) in print("Delete: \(indexPath.row + 1)") completionHandler(true) } // swipe let swipe = UISwipeActionsConfiguration(actions: [delete]) return swipe }
Trong đó:
delete
là action chúng ta khai báo vớititle
vàstyle
- Sự kiện nhận được khi thao tác thì gọi closure với các tham số
action, view, completion
- Chú ý
completion
để kết thúc tác vụ và update giao diện trên TableView
Kết quả như sau khi bạn vuốt từ phải sang trái
Tiếp tục, bạn thêm một ít màu mè vào cho đẹp. Tham khảo đoạn code sau:
let delete = UIContextualAction(style: .normal, title: "Delete") { (action, view, completionHandler) in print("Delete: \(indexPath.row + 1)") completionHandler(true) } delete.image = UIImage(systemName: "trash") delete.backgroundColor = .red
Kết quả như sau:
3. Trailing Swipe
Đây là tác vụ khi người dùng vuốt từ phải sang trái. Như đã được đề cập ở các phần trên thì chúng ta có thể thêm nhiều action. Tham khảo đoạn code sau:
func tableView(_ tableView: UITableView, trailingSwipeActionsConfigurationForRowAt indexPath: IndexPath) -> UISwipeActionsConfiguration? { // delete let delete = UIContextualAction(style: .normal, title: "Delete") { (action, view, completionHandler) in print("Delete: \(indexPath.row + 1)") completionHandler(true) } delete.image = UIImage(systemName: "trash") delete.backgroundColor = .red // share let share = UIContextualAction(style: .normal, title: "Share") { (action, view, completionHandler) in print("Share: \(indexPath.row + 1)") completionHandler(true) } share.image = UIImage(systemName: "square.and.arrow.up") share.backgroundColor = .blue // download let download = UIContextualAction(style: .normal, title: "Download") { (action, view, completionHandler) in print("Download: \(indexPath.row + 1)") completionHandler(true) } download.image = UIImage(systemName: "arrow.down") download.backgroundColor = .green // swipe let swipe = UISwipeActionsConfiguration(actions: [delete, share, download]) return swipe }
Trong đó, mình sử dụng 3 action (delete, share và download) nằm ẩn trong cell. Khi nhận được sự kiện vuốt từ phải sang trái, thì chúng sẽ xuất hiện. Xem kết quả như sau:
4. Leading Swipe
Bạn sẽ khá là thích thú khi có thể làm điều ngược lại là vuốt từ trái sang phải. Woà, lúc này, chúng ta có thể tiết kiện đi khá nhiều diện tích trên cell. Các tính năng được ẩn đi từ 2 phái của cell.
Bạn tham khảo đoạn code sau:
func tableView(_ tableView: UITableView, leadingSwipeActionsConfigurationForRowAt indexPath: IndexPath) -> UISwipeActionsConfiguration? { // favorite let favorite = UIContextualAction(style: .normal, title: "favorite") { (action, view, completionHandler) in print("favorite: \(indexPath.row + 1)") completionHandler(true) } favorite.image = UIImage(systemName: "suit.heart.fill") favorite.backgroundColor = .systemPink // profile let profile = UIContextualAction(style: .normal, title: "profile") { (action, view, completionHandler) in print("profile: \(indexPath.row + 1)") completionHandler(true) } profile.image = UIImage(systemName: "person.fill") profile.backgroundColor = .yellow // report let report = UIContextualAction(style: .normal, title: "report") { (action, view, completionHandler) in print("report: \(indexPath.row + 1)") completionHandler(true) } report.image = UIImage(systemName: "person.crop.circle.badge.xmark") report.backgroundColor = .lightGray // swipe let swipe = UISwipeActionsConfiguration(actions: [profile, favorite, report]) return swipe }
Xem kết quả như sau:
OKAY! Qua vài đường cơ bản thì bạn có thể sử dụng thêm nhiều actions cho cell của bạn. Không lo việc ảnh hưởng tới giao diện và không làm thay đổi cấu trúc re-useable
của TableView.
Mình xin kết thúc bài hướng dẫn này tại đây. Và bạn có thể xem lại bài hướng dẫn thông qua video demo. Ngoài ra, bạn có thể checkout code tại đây.
Tạm Kết
- Các loại Swipe Actions trong UITableViewCell
- Tạo và custom một Action
- Sử dụng được 2 sự kiện vuốt (trailing & leading)
- Ẩn đi và sử dụng được nhiều tính năng hơn
Nếu thay hay thì like, share và subscribe channel … để nhiều người cùng theo dõi. Cảm ơn bạn đã đọc bài viết này!
Related Posts:
Written by chuotfx
Hãy ngồi xuống, uống miếng bánh và ăn miếng trà. Chúng ta cùng nhau đàm đạo về đời, về code nhóe!
Leave a Reply Cancel reply
Fan page
Tags
Recent Posts
- Mô phỏng chiến lược SNOWBALL giúp AI “Nhớ Lâu” hơn trong cuộc trò chuyện
- Prompt for Coding – Bug Detection với prompting cơ bản
- Cẩm Nang Đặt Câu Hỏi Chain of Verification (CoVe): Từ Cơ Bản Đến Chuyên Gia
- Chain of Verification (CoVe): Nâng Cao Độ Tin Cậy Của Mô Hình Ngôn Ngữ Lớn
- Mixture of Thought (MoT) – Từ Suy Luận Logic đến Ứng Dụng Sáng Tạo
- Prompt Injection (phần 2) – Chiến Lược Phòng Thủ và Kỹ Thuật Giảm Thiểu Rủi Ro
- Prompt Injection (phần 1) – Phân Tích về Các Kỹ Thuật Tấn Công
- Bản chất của Prompt Engineering là Quản lý sự Mơ hồ
- Inject Marker : Từ Chỉ Dẫn Đơn Giản Đến Công Cụ Khoa Học Để Làm Chủ AI
- Prompt Engineering – Người lập trình ngôn ngữ tự nhiên
You may also like:
Archives
- July 2025 (10)
- June 2025 (1)
- May 2025 (2)
- April 2025 (1)
- March 2025 (8)
- January 2025 (7)
- December 2024 (4)
- September 2024 (1)
- July 2024 (1)
- June 2024 (1)
- May 2024 (4)
- April 2024 (2)
- March 2024 (5)
- January 2024 (4)
- February 2023 (1)
- January 2023 (2)
- November 2022 (2)
- October 2022 (1)
- September 2022 (5)
- August 2022 (6)
- July 2022 (7)
- June 2022 (8)
- May 2022 (5)
- April 2022 (1)
- March 2022 (3)
- February 2022 (5)
- January 2022 (4)
- December 2021 (6)
- November 2021 (8)
- October 2021 (8)
- September 2021 (8)
- August 2021 (8)
- July 2021 (9)
- June 2021 (8)
- May 2021 (7)
- April 2021 (11)
- March 2021 (12)
- February 2021 (3)
- January 2021 (3)
- December 2020 (3)
- November 2020 (9)
- October 2020 (7)
- September 2020 (17)
- August 2020 (1)
- July 2020 (3)
- June 2020 (1)
- May 2020 (2)
- April 2020 (3)
- March 2020 (20)
- February 2020 (5)
- January 2020 (2)
- December 2019 (12)
- November 2019 (12)
- October 2019 (19)
- September 2019 (17)
- August 2019 (10)